If you are building a website or web application using PHP, it’s common to need to redirect users from one page to another. This could be for a variety of reasons, such as after a successful login or after submitting a form.
In this article, we will explore how to redirect to another page in PHP, covering both header redirects and JavaScript redirects.
Table of Contents
Introduction
Redirecting to another page in PHP is a common task, and there are multiple ways to achieve it. In this article, we will cover two popular methods for redirecting: header redirects and JavaScript redirects. Both methods have their pros and cons, so we’ll discuss which one is best for different scenarios.
What is Redirect in PHP
In PHP, a redirect is a way to send a response to the browser that instructs it to go to a different web page. Redirects are commonly used when a user submits a form, logs in, or performs some other action that requires a change in the current page.
Why is it Important to Handle a 404 Error Properly?
Handling a 404 error properly is important for several reasons:
User Experience
When a user encounters a 404 error, it interrupts their browsing experience and may lead them to leave your website. Providing a custom error page or redirecting them to a relevant page can help retain their interest and improve their overall experience.
Search Engine Optimization (SEO)
Search engines, such as Google, consider 404 errors as a negative signal, which can negatively impact your website’s search engine rankings. Handling 404 errors properly by redirecting to relevant content can help preserve your website’s SEO efforts.
Crawl Efficiency
When a search engine crawls your website and encounters a 404 error, it may continue to crawl other pages on your website. However, if it encounters too many 404 errors, it may assume that your website is poorly maintained and may decrease its crawl frequency.
How To Redirect To Another Page In PHP
Header Redirects
A header redirect is a server-side redirect that sends a header to the browser, instructing it to load a new page. Here’s how to do it in PHP:
Using the header() Function
The header() function is a built-in PHP function that allows you to send HTTP headers. To redirect to another page, you can use the Location header with the URL of the page you want to redirect to:
header('Location: http://example.com/new-page.php'); exit;
Note that the exit statement is important, as it ensures that no further code is executed on the current page after the redirect header is sent.
Redirecting with a Delay
If you want to delay the redirect by a few seconds, you can use the sleep() function to pause execution before sending the header:
sleep(3); header('Location: http://example.com/new-page.php'); exit;
This will delay the redirect by three seconds.
Meta Refresh Redirects
Meta refresh redirects use a special HTML tag to instruct the browser to refresh the current page after a certain amount of time. For example:
<meta http-equiv="refresh" content="5;url=http://example.com/new-page.php">
This will refresh the current page after 5 seconds and redirect the browser to the URL “http://example.com/new-page.php”.
Redirects are useful for maintaining the flow of a user’s experience on a website, and can also be used for SEO purposes to redirect old or broken URLs to new ones.
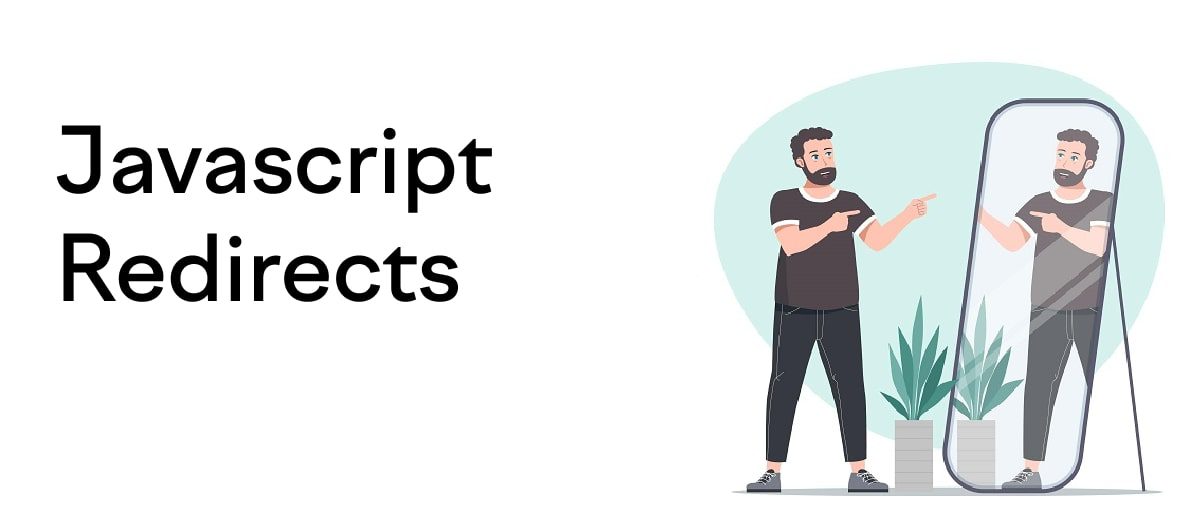
JavaScript Redirects
A JavaScript redirect is a client-side redirect that uses JavaScript code to load a new page. Here’s how to do it in PHP:
Using the window.location Object
The window.location object is a built-in JavaScript object that contains information about the current URL and allows you to navigate to a new URL. To redirect to another page, you can set the window.location.href property to the URL of the page you want to redirect to:
echo '<script>window.location.href = "http://example.com/new-page.php";</script>';
Redirecting with a Delay
To delay the redirect using JavaScript, you can use the setTimeout() function to execute the redirect after a certain amount of time:
echo '<script>setTimeout(function() { window.location.href = "http://example.com/new-page.php"; }, 3000);</script>';
This will delay the redirect by three seconds.
Redirect Based on User Agent
You can use conditional statements to redirect based on user agent, IP address, or other criteria. For example:
if (strpos($_SERVER['HTTP_USER_AGENT'], 'MSIE') !== FALSE) { header('Location: http://example.com/new-page-ie.php'); } else { header('Location: http://example.com/new-page.php'); } exit;
This will redirect users with Internet Explorer to a different page than users with other browsers.
Redirecting to the Custom 404 Page
The final step is to redirect the user to the custom 404 page when a 404 error is encountered. This can be done using the .htaccess file or through PHP code.
Using .htaccess
To redirect users to a custom 404 page using .htaccess, add the following code to your file:
ErrorDocument 404 /404.php
Make sure to replace /404.php with the URL of your custom 404 page.
Using PHP Code
To redirect users to a custom 404 page using PHP, add the following code to your PHP file:
header("HTTP/1.0 404 Not Found"); include("404.php"); exit();
Make sure to replace 404.php with the filename of your custom 404 page.
Choosing Between Header and JavaScript Redirects
Both header and JavaScript redirects have their pros and cons. Header redirects are faster and more reliable, as they are handled by the server and do not rely on JavaScript support in the browser.
However, they cannot be delayed or customized with animations or other effects. JavaScript redirects, on the other hand, offer more flexibility and can be customized with animations or other effects, but they are slower and less reliable, as they rely on JavaScript support in the browser.
When deciding which method to use, consider your specific needs and the trade-offs between speed, reliability, and customization.
Common Redirect Errors and How to Fix Them
There are a few common errors that can occur when redirecting in PHP, such as “headers already sent” and “redirect loop”. Here’s how to fix them:
Headers Already Sent
This error occurs when there is output sent to the browser before the header() function is called. To fix it, make sure there is no output before the header() function, and use the ob_start() function to buffer output:
ob_start(); header('Location: http://example.com/new-page.php'); ob_end_flush(); exit;
his will buffer the output until the script is finished, ensuring that no output is sent before the header() function is called.
Redirect Loop
This error occurs when the same page is redirected repeatedly, creating an infinite loop. To fix it, make sure that the redirect target is different from the current page, and check for any conditions that might cause the redirect to occur repeatedly. How To Redirect To Another Page In HTML.
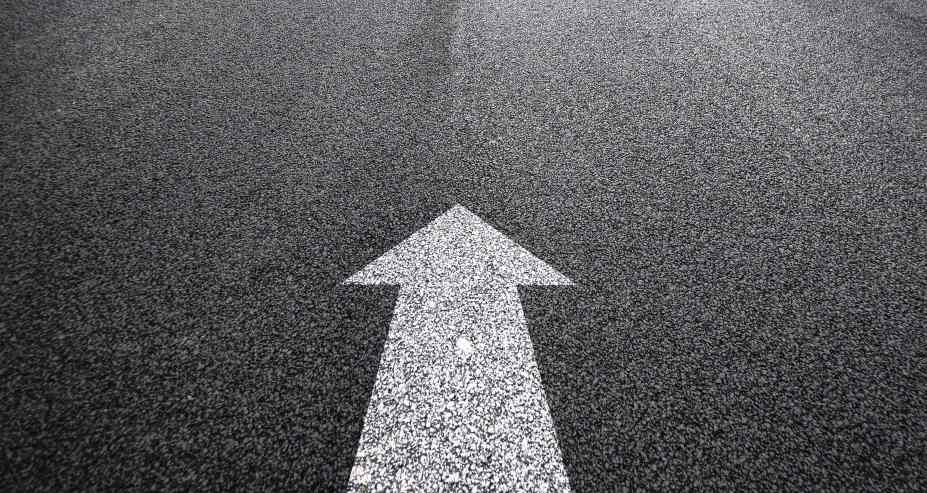
Best Practices for Handling 404 Errors
Now that you know how to implement a PHP 404 redirect, here are some best practices to ensure that your website’s visitors have a seamless browsing experience and search engines can crawl your website efficiently:
Keep the User in Mind
When creating a custom 404 page, keep the user in mind. Make sure to provide useful information, such as a clear message that the requested page is not available, and offer alternative links or a search bar to help the user continue browsing your website.
Monitor 404 Errors
Regularly monitor your website’s 404 errors using tools such as Google Search Console or third-party tools. This will help you identify any patterns or trends and quickly address any issues.
Redirect to Relevant Content
When redirecting users from a 404 error page, make sure to redirect them to relevant content. For example, if the user was trying to access a product page that no longer exists, redirect them to a similar product or a relevant category page.
Update Your Sitemap
Make sure to regularly update your website’s sitemap to include any new pages or remove any deleted pages. This will help search engines crawl your website efficiently and reduce the number of 404 errors.
Fix Broken Links
Regularly check for broken links on your website using tools such as Broken Link Checker or Google Search Console. Fixing broken links can help reduce the number of 404 errors and improve your website’s user experience.
FAQs
Can I redirect to a relative URL instead of an absolute URL?
Yes, you can use a relative URL with the header() function.
Do I need to use exit() after the header() function?
Yes, it’s important to use exit() after the header() function, as it ensures that no further code is executed on the current page after the redirect header is sent.
Can I customize the delay or animation of a header redirect?
No, header redirects cannot be delayed or customized with animations or other effects. If you need to customize the redirect, consider using a JavaScript redirect instead.
Why am I getting a “headers already sent” error?
This error occurs when there is output sent to the browser before the header() function is called. To fix it, make sure there is no output before the header() function, and use the ob_start() function to buffer output.
Why am I getting a “redirect loop” error?
This error occurs when the same page is redirected to repeatedly, creating an infinite loop. To fix it, make sure that the redirect target is different from the current page. Check for any conditions that might cause the redirect to occur repeatedly.
Conclusion
Redirecting to another page in PHP is a common task that can be achieved using either header redirects or JavaScript redirects. Both methods have their pros and cons, so it’s important to consider your specific needs when choosing which one to use.
By following the guidelines and best practices outlined in this article, you can ensure that your redirects are fast, reliable, and error-free.
- NordVPN New Sales 30% OFF!!!
- Ultimate Photography Bundle for new users
- Get 4 Months Free on All Shared Hosting Plans With Code, wpe4free. Buy now!
Save 35% On Tools Tailored For Your Amazon Business
More Redirects Posts
If you’re looking for more redirects post, check out our following post:
- How To Redirect To Another Page In HTML
- When Would It Be Necessary To 301 Redirect?
- 11 Best WP 301 Redirect Plugins For Improved SEO
- Best 404 Redirect WordPress Plugin: Everything You Need to Know
- How To Make A Redirect Link In WordPress: A Step-by-Step Guide
- How To Install Link Redirect Trace On Firefox And Chrome
- What Does 404 Page Not Found Mean? Understand The Error
- 301 Redirect In PHP: The Key To Maintaining Your Website’s SEO